Normally the items in a CheckedListBox are hardcoded or added programmatically (from an enum or from binding a data source). But sometimes you may want to allow the user to add and remove items from a CheckedListItem.
In this article I’ll show how to add items and remove them. I’ll be working with text, and will be using the following form:
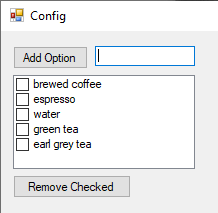
Add items to the CheckedListBox
You add items by calling list.Items.Add() like this:
private void btnAdd_Click(object sender, EventArgs e)
{
listOptions.Items.Add(txtOption.Text);
}
Code language: C# (cs)
Remove items from the CheckedListBox
To remove checked items, you have to loop in reverse and call RemoveAt(index), like this:
private void btnRemove_Click(object sender, EventArgs e)
{
for (int i = listOptions.Items.Count - 1; i >= 0; i--)
{
if (listOptions.GetItemChecked(i))
listOptions.Items.RemoveAt(i);
}
}
Code language: C# (cs)
Can’t loop forward and remove items
When removing items from a list in a loop, you need to loop in reverse. When you remove an item, it changes the index of the items following that removed item. This leads to incorrect results.
As an example of why you can’t loop forward to remove items, consider the following incorrect code:
var ints = new List<int> { 1, 2, 3, 4, 5 };
for(int i = 0; i < ints.Count; i++)
{
ints.RemoveAt(i);
}
Code language: C# (cs)
You may think this would remove all items in the list, but it skips over items due to the index of those items changing. At the end, the list still has { 2, 4 }.
When you loop in reverse and remove items, it changes the index of the items that you already looped over, so there’s no side effects.
Can’t use a foreach
You may be thinking, why not use a foreach on list.CheckedItems to remove items? Like this:
foreach(var item in listOptions.CheckedItems)
{
listOptions.Items.Remove(item);
}
Code language: C# (cs)
But you can’t modify a list you’re looping over it with a foreach, otherwise you get a runtime exception (InvalidOperationException).