A CheckedListBox allows the user to check one or more checkboxes. Sometimes you’ll want to be able to check the boxes programmatically. For example, you may want to allow the user to check or uncheck all boxes at once. Or perhaps you want to persist the values the user checked and load them later.
To programmatically check a box, you can use either of the following methods:
checkedListBox.SetItemChecked(0, true);
checkedListBox.SetItemCheckState(0, CheckState.Checked);
Code language: C# (cs)
In this article, I’ll show examples of how to check / uncheck all boxes at once, and how to load previously selected values. I’ll use the following WinForm:
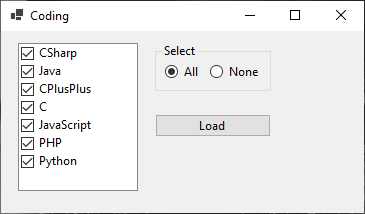
Initialize the CheckedListBox
First, add an enum with the [Flags] attribute. This simplifies things if your goal is to persist the selected values and load them later.
[Flags]
public enum ProgrammingLanguages
{
CSharp = 1,
Java = 2,
CPlusPlus = 4,
C = 8,
JavaScript = 16,
PHP = 32,
Python = 64
}
Code language: C# (cs)
Next, initialize the CheckedListBox in the form constructor, like this:
public frmCoding()
{
InitializeComponent();
clbLangs.Items.AddRange(Enum.GetNames(typeof(ProgrammingLanguages)));
}
Code language: C# (cs)
Checking or unchecking all checkboxes
To check or uncheck all checkboxes at once, you loop through the checkboxes and call SetItemCheckState(), like this:
private void rbSelectAll_CheckedChanged(object sender, EventArgs e)
{
SetAllCheckboxes(CheckState.Checked);
}
private void rbSelectNone_CheckedChanged(object sender, EventArgs e)
{
SetAllCheckboxes(CheckState.Unchecked);
}
private void SetAllCheckboxes(CheckState checkState)
{
for (int i = 0; i < clbLangs.Items.Count; i++)
{
clbLangs.SetItemCheckState(i, checkState);
}
}
Code language: C# (cs)
Read more about how to get the selected values in a CheckedListBox.
Loading previously checked values
Let’s say you saved the checked values to the database, and you want to load the checked values when the user clicks a button.
To do that, you can loop through the enum values and set the checked state based on the result of HasFlag(), like this:
private void btnLoad_Click(object sender, EventArgs e)
{
var programmer = new Programmer()
{
Langs = ProgrammingLanguages.CSharp | ProgrammingLanguages.Java | ProgrammingLanguages.Python
};
var langs = Enum.GetValues(typeof(ProgrammingLanguages)) as ProgrammingLanguages[];
for (int i = 0; i < langs.Count(); i++)
{
clbLangs.SetItemChecked(i, programmer.Langs.HasFlag(langs[i]));
}
}
Code language: C# (cs)
Clicking the load button will check the CSharp, Java, and Python checkboxes and leave all the other checkboxes unchecked.