When you need to show enum values in a dropdown (ComboBox control with DropDownStyle=DropDown), it’s a good idea to automatically populate the list, instead of manually setting all of the values.
To fill the dropdown with the enum’s values, set the DataSource to Enum.Values(), like this:
dropDownListPets.DataSource = Enum.GetValues(typeof(PetType));
Code language: C# (cs)
Read more about how to show the enum’s Description attribute instead of the enum name.
Then to get the option the user picked, do the following:
var choice = (PetType)dropDownListPets.SelectedItem;
Code language: C# (cs)
When I launch my form, I can see it correctly populated the dropdown from my Pets enum:
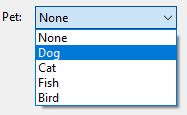
Binding to an enum property
Let’s say you’re binding your controls to an object, and one of the object’s properties is an enum. Here’s an example of how to bind the enum property to a dropdown control:
person = new Person()
{
Name = "Bob",
Pet = PetType.Dog
};
//auto-populate with all possible values
dropDownListPets.DataSource = Enum.GetValues(typeof(PetType));
//bind the enum property
dropDownListPets.DataBindings.Add(nameof(ComboBox.SelectedItem), person, nameof(person.Pet));
Code language: C# (cs)