Problem
You’re using a DataGridView and you can’t edit one or more of the columns. When you click a cell, it just highlights and doesn’t enter editing mode.
Check if your grid, column, or bound property are readonly. If any of these are readonly, you won’t be able to edit the values in the column.
Solution
Case 1 – DataGridView is readonly
The first thing to do is to check if the grid is readonly. If it’s readonly, you won’t be able to edit any of the columns. Set the grid’s ReadOnly property to false.
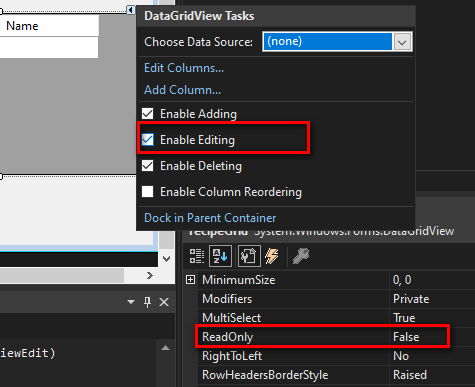
Note: Checking ‘Enable Editing’ does the same thing (it links to the ReadOnly property).
If that looks fine, check if your code is programmatically setting the ReadOnly somewhere.
Case 2 – Column is readonly
If you added the column manually through the UI or programmatically (instead of columns getting generated automatically), then check if the column is readonly. Set the column’s ReadOnly property to false.
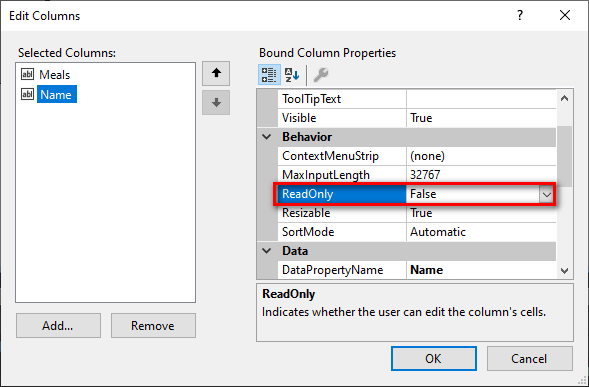
Check the code to see if you’re setting the ReadOnly property anywhere.
Case 3 – Column is bound to a property with a non-public setter
In order to make a column editable, it needs to be bound to a property with a public setter. If the property has a non-public setter, it effectively makes the column readonly.
Here’s an example. Let’s say you’re binding the DataGridView to a list of recipes, and the Recipe class has the following definition:
public class Recipe
{
public string Name { get; set; }
public int Meals { get; internal set; }
}
Code language: C# (cs)
The Meals property has a non-public setter. Therefore the Meals column will be readonly and you won’t be able to edit it. Make the setter public:
public class Recipe
{
public string Name { get; set; }
public int Meals { get; set; }
}
Code language: C# (cs)
Case 4 – Some event handler is preventing the edit from committing
If you still can’t edit the column, then check if you have an event handler that is interfering with edits.
Here’s just one of many possible examples. Let’s say you have the following CellValidating event handler, which conditionally calls CancelEdit():
private void recipeGrid_CellValidating(object sender, DataGridViewCellValidatingEventArgs e)
{
//some conditional logic
recipeGrid.CancelEdit();
}
Code language: C# (cs)
Let’s say the conditional logic is faulty, such that it always results in it calling CancelEdit(). In this scenario, you’d be able to enter new values, but it’ll always revert to the old value. In other words, you can enter editing mode, but you can’t edit.