To disable a test temporarily, you can add the [Ignore] attribute to the test method, like this:
[TestMethod()]
[Ignore]
public void SpeedTest()
{
//the test
}
Code language: C# (cs)
When you add the [Ignore] attribute, the test will be ignored by the test runner. It will show up in Test Explorer with a warning icon and will count as Skipped.
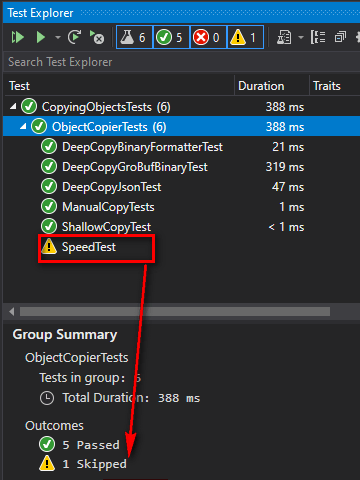
Note: This isn’t the same as the unit test not running unexpectedly (which shows up as ‘inconclusive’).
Why would you want to disable a unit test?
There are many reasons why you might want to disable a unit test temporarily. The key word here is temporarily. If a test is bad or no longer makes sense, just delete it.
However, if a test is good, and you just don’t want to run it right now, then disabling it is a pragmatic choice.
You may be asking, why can’t I just comment out the test instead? By adding the [Ignore] attribute, the test shows up in test results. It doesn’t run, and is counted as Skipped. It’s very hard to forget about this test, because this warning symbol in the test results is a constant reminder that you have a disabled test. If you were to simply comment out the test, you’d very likely forget about it and it’d stay commented out forever. If this test had any value to begin with, then that would be a bad thing.
Here are three specific reasons why you might want to disable a test. You may have your own reasons.
1 – The test fails for the wrong reasons
After a code change, the test now has invalid assumptions, and fails for the wrong reasons (or perhaps the unit test fails and stops all other tests from running). You’ll have to update the test’s assumptions to get it passing, but you don’t have time to fix it at this moment.
2 – The test is very slow
One of the key benefits of unit tests is that they run fast and act as a rapid feedback loop while you’re developing. If running all of the tests is slow, then people won’t run the tests at all. It only takes one slow test to discourage people from running all of the tests (“bad one apple ruins the batch”).
A test could be slow for many reasons, but for now, you simply want to disable it so you can easily run all of the other tests without being slowed down by this one test.
3 – You only want to run this test on-demand, not automatically
You might have integration tests mixed in with your unit tests. Or one of your tests might not be a test at all. Sometimes I like to add performance tests (let’s see how fast algorithm A is compared to algorithm B) in unit test projects for convenience.
In any case, the point is you have tests that you want to only run manually on-demand. Therefore it makes sense to use the [Ignore] attribute on these tests.