When you need to let the user select a date and/or time of day, you can use the DateTimePicker control:
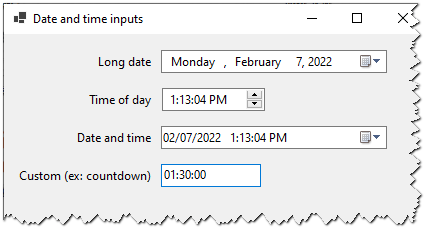
You can set the control properties in the UI or programmatically:
public frmDateTime()
{
InitializeComponent();
longDatePicker.Format = DateTimePickerFormat.Long;
longDatePicker.Value = new DateTime(year: 2025, month: 1, day: 1);
}
Code language: C# (cs)
If you don’t set an initial value, it’ll default to DateTime.Now (at the time the code is executed).
The value the user picked is available via the DateTimePicker.Value property. This is a DateTime object.
private void btnSave_Click(object sender, EventArgs e)
{
DateTime longDate = longDatePicker.Value;
//save to the database etc...
}
Code language: C# (cs)
To show the DateTime as a string in the UI, you can use either the built-in format specifiers – Long (date), Short (date), Time – or use a custom format. If you use one of the built-in format specifiers, it’ll use the current system’s date/time format. I’ll show examples of using the Time format to get the time of day, using a custom format to get the date and time, and using a different control for getting non-clock time.
Table of Contents
Time of day
Here’s an example of using the Time format to get the time of day. It displays just the time and uses the up/down picker:
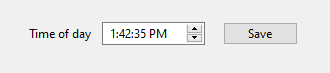
Here’s how to use the Time format and make it use the up/down picker:
public frmDateTime()
{
InitializeComponent();
timeOfDayPicker.Format = DateTimePickerFormat.Time;
timeOfDayPicker.ShowUpDown = true;
}
Code language: C# (cs)
Note: DateTimePicker has two types of pickers – calendar and up/down. The up/down picker is good for when you’re only displaying the time of day.
You can set the initial time of day value with a DateTime. This is a bit awkward, because you have to set the date part of the DateTime (just use 2000-1-1):
timeOfDayPicker.Value = new DateTime(2000, 1, 1, hour: 11, minute: 0, second: 0);
Code language: C# (cs)
Here’s how to get the value:
private void btnSave_Click(object sender, EventArgs e)
{
DateTime timeOfDay = timeOfDayPicker.Value;
//save to the database etc...
}
Code language: C# (cs)
Date and time
Here’s an example of using a custom format to allow the user to select both the date and time. It’s using the calendar picker:

You have to tell it you’re using a custom format and also set the custom format string:
public frmDateTime()
{
InitializeComponent();
dateTimePicker.Format = DateTimePickerFormat.Custom;
dateTimePicker.CustomFormat = "MM/dd/yyyy h:mm:ss tt";
}
Code language: C# (cs)
You can set the initial value to any DateTime object. Here’s an example of defaulting it to 2022-02-07 14:00:00:
dateTimePicker.Value = new DateTime(year: 2022, month: 2, day: 7, hour: 14, minute: 0, second: 0);
Code language: C# (cs)
Get the selected DateTime from the Value property:
private void btnSave_Click(object sender, EventArgs e)
{
DateTime dateTime = dateTimePicker.Value;
//save to the database etc...
}
Code language: C# (cs)
Non-clock time
DateTimePicker is good for time of day input – i.e. a specific time on the clock. For other types of time input – such as duration of time – you can either use a MaskedTextBox or a NumericUpDown control.
Use NumericUpDown when you only need to get one unit of time (ex: just hours). Otherwise use MaskedTextBox to handle the more complex scenario of accepting multiple units of time (ex: hours and minutes).
Here’s an example of using MaskedTextBox. Let’s say you want the user to input a countdown time in hours, minutes, and seconds:

Configure the custom format by setting the MaskedTextBox.Mask property, and set a good default value.
public frmDateTime()
{
InitializeComponent();
countdownTimerPicker.Mask = "00:00:00";
countdownTimerPicker.Text = "01:30:00";
}
Code language: C# (cs)
Note: 00:00:00 means they can only select digits (0-9) for all positions.
Get the input from MaskedTextBox.Text and parse a DateTime from this string based on your custom format. For example, this is parsing the 00:00:00 formatted string into a TimeSpan:
private void btnSave_Click(object sender, EventArgs e)
{
var timeParts = countdownTimerPicker.Text.Split(":");
int hours = Convert.ToInt32(timeParts[0]);
int minutes = Convert.ToInt32(timeParts[1]);
int seconds = Convert.ToInt32(timeParts[2]);
var timeSpan = new TimeSpan(hours, minutes, seconds);
MessageBox.Show($"Hours = {timeSpan.TotalHours}");
}
Code language: C# (cs)
This parses the “01:30:00” string into a TimeSpan and outputs the total hours as the following:
Hours = 1.5
Code language: plaintext (plaintext)