Problem
I’m trying to use Selenium with the ChromeDriver and I’m running into the following exception:
Unhandled Exception: OpenQA.Selenium.DriverServiceNotFoundException: The chromedriver.exe file does not exist in the current directory or in a directory on the PATH environment variable. The driver can be downloaded at http://chromedriver.storage.googleapis.com/index.html
Solution
The simplest solution is to install the Selenium.Chrome.WebDriver package:
Install-Package Selenium.Chrome.WebDriver
Code language: PowerShell (powershell)
Note: This is using Package Manager Console (View > Other Windows > Package Manager Console).
This results in the ChromeDriver.exe being put into your build directory.
Note: You can always specify the chromedriver.exe in the constructor, but this requires you to have it installed already. Installing via nuget is a simpler approach.
Manually downloading the right chromedriver
Problem – chromedriver version incompatibility
When you attempt to use a version of chromedriver that’s incompatible with the browser you’re targeting, you’ll run into error messages like the following:
System.InvalidOperationException: session not created: Chrome version must be between 70 and 73
(Driver info: chromedriver=2.45.615291
System.InvalidOperationException: session not created: This version of ChromeDriver only supports Chrome version 85 (SessionNotCreated)
Solution – download the right chromedriver manually
At the current time, the latest version of Chrome is v89, and the Selenium.Chrome.WebDriver nuget package currently doesn’t contain the latest version.
Instead of getting the chromedriver from Selenium.Chrome.WebDriver, you can manually download whatever chromedriver version you need and add it to your project.
- Download the right chromedriver.exe version you need from Chromium ChromeDriver downloads.
- Add chromedriver.exe into your project and make it output to the build directory:
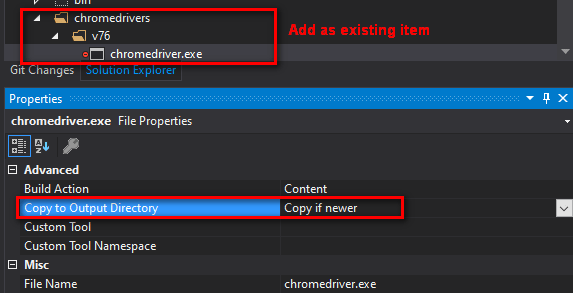
- Specify the chromedriver directory by passing in the chromeDriverDirectory, like this:
var options = new ChromeOptions();
options.BinaryLocation = @"C:\Chrome\v76\chrome.exe";
IWebDriver driver = new ChromeDriver(chromeDriverDirectory: @"chromedrivers\v76", options);
driver.Navigate().GoToUrl("https://www.google.com");
Code language: C# (cs)
Note: You can deal with multiple versions of Chrome / ChromeDriver at the same time using this approach.
I have mine setup thru Nuget however have a number of tests I want to do and utilize various versions of Chrome. So I was able to create calls with:
options.BinaryLocation = @”C:\temp\GoogleChrome_87\App\Chrome-bin\chrome.exe”;
To a portable version of Chrome. However then Chromedriver craps out that it’s not compatible with this version as the version installed thru Nuget is Chromedriver 89. So I use Chromedriver 89 for at least one scenario, but how would I add a argument or whatever it would be for the to point to a separate chromedriver.exe that is from version 87?
Hi John,
You can accomplish that by manually downloading the specific versions you need. You can include the multiple chromedriver.exe’s in your project and output them to the build directory, and then use the different versions like the code below. Please take a look at the new section I added to the article called Manually downloading the right chromedriver.
var options89 = new ChromeOptions();
//this is the version I have installed, so don't need to set BinaryLocation explicitly
IWebDriver chrome89 = new ChromeDriver(chromeDriverDirectory: @"chromedrivers\v89" , options89);
chrome89.Navigate().GoToUrl("https://www.google.com");
var options76 = new ChromeOptions();
options76.BinaryLocation = @"C:\Chrome\v76\chrome.exe";
IWebDriver chrome76 = new ChromeDriver(chromeDriverDirectory: @"chromedrivers\v76", options76);
chrome76.Navigate().GoToUrl("https://www.google.com");
Note: Make sure to put the different chromedriver.exe’s into versioned folders, like:
/chromedrivers/v76/chromedriver.exe
/chromedrivers/v89/chromedriver.exe