The top-level statements feature makes the Main() method implicit. This feature was added in C# 9 (.NET 5) with the purpose of decluttering a project’s entry point.
Here’s what a console app looks like using a top-level statement:
Console.WriteLine("Hello, World!");
Code language: C# (cs)
The compiler generates the Main() method implicitly from the top-level statements. The code above is functionally equivalent to the following console app with an explicit Main() method:
namespace ConsoleAppWithMain
{
internal class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello, World!");
}
}
}
Code language: C# (cs)
Everything is implicit with top-level statements. This can make it a little confusing to figure out how to do the usual things you’d do in Main(), such as the following:
- Get and parse arguments passed in by the user when the executable is started (via ‘args’).
- Use async/await.
- Return an exit code.
Simply put, you can just do them. Here’s an example of doing all these things with top-level statements:
if (args.Length == 1)
{
int delay = Convert.ToInt32(args[0]);
Console.WriteLine($"Delaying {delay} seconds");
await Task.Delay(TimeSpan.FromSeconds(delay));
return 0;
}
else
{
Console.WriteLine("No arguments passed in");
return 1;
}
Code language: C# (cs)
Note: The implicit ‘args’ variable doesn’t show up in IntelliSense, but it’s there. Use it just like you would any other string array, and ignore the initial compiler error.
Running this console with a valid argument results in the following output:
Delaying 1 seconds
ConsoleApp.exe (process 27844) exited with code 0.
Code language: plaintext (plaintext)
Opting out of using top-level statements
Projects created in .NET 6+ use the top-level statements feature by default. You can opt out of using this if by checking the Do not use top-level statements checkbox during project creation:
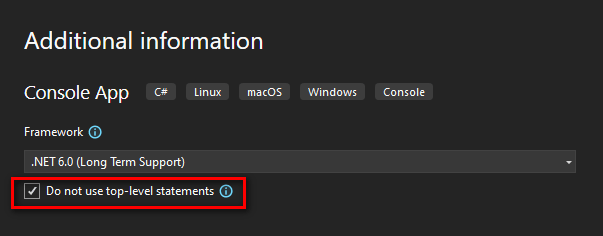
It’ll create the project with an explicit Main() method.
Note: The top-level statements feature was added in .NET 5, but it wasn’t until .NET 6 that it was put into project templates and became the default.
Converting an existing project to use a top-level statement
You can use a built-in refactoring command to convert an existing project (on .NET 5+) to use top-level statements.
- In the source file containing the Main() method, click on the quick tips lightbulb.
- Click Convert to top-level statements.
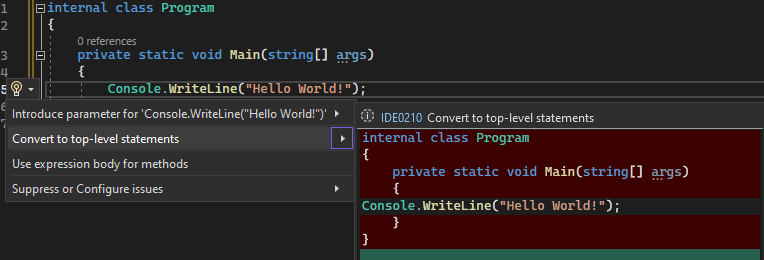
Note: You can do this refactoring manually, but you might as well use the built-in refactoring command.
You can do the reverse conversion (Main() to top-level statements) by using a refactoring command as well.