The simplest way to check if a directory is empty is by calling Directory.EnumerateFileSystemEntries(). If this doesn’t return anything, then the directory doesn’t have any files or subdirectories (folders), which means it’s empty. Here’s an example:
using System.IO;
using System.Linq;
if (!Directory.EnumerateFileSystemEntries(@"C:\Data\").Any())
{
Console.WriteLine("Directory is empty");
}
else
{
Console.WriteLine("Directory is not empty");
}
Code language: C# (cs)
Another situation to consider is when a directory has no files but might have empty subdirectories (folders). You can check this by calling Directory.EnumerateFiles() with SearchOption.AllDirectories. This searches the directory for files recursively. If it doesn’t return anything, then the directory doesn’t have any files and is empty. Here’s an example:
using System.IO;
using System.Linq;
if (!Directory.EnumerateFiles(@"C:\Data\", "*", SearchOption.AllDirectories).Any())
{
Console.WriteLine("Directory is empty");
}
else
{
Console.WriteLine("Directory is not empty");
}
Code language: C# (cs)
Find all empty directories
Instead of just checking if a directory is empty, let’s say you want to find all empty directories and output their paths. To do this, recursively search through subdirectories for files. Use Directory.EnumerateDirectories() to loop through subdirectories and Directory.EnumerateFiles() to check for files. Here’s an example:
using System.IO;
using System.Linq;
bool IsDirectoryEmpty(string path)
{
bool subDirectoriesAreEmpty = true;
foreach (var subDir in Directory.EnumerateDirectories(path))
{
if (IsDirectoryEmpty(subDir))
{
Console.WriteLine($"Empty: {subDir}");
}
else
{
subDirectoriesAreEmpty = false;
}
}
if (subDirectoriesAreEmpty && !Directory.EnumerateFiles(path).Any())
{
return true;
}
return false;
}
Code language: C# (cs)
Note: You can use this as a starting point for dealing with empty directories however you need to. This is simply outputting the paths to the console.
Now I’ll show an example of running this code against a directory – C:\temp\EmptyTest\ – with the following structure:
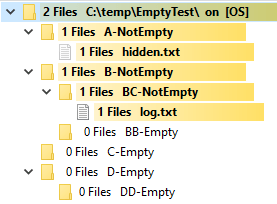
As you can see, this directory contains four empty subdirectories. Here’s how to run the code against this directory:
string rootPath = @"C:\temp\EmptyTest\";
Console.WriteLine($"Finding all empty directories in {rootPath}");
if (IsDirectoryEmpty(rootPath))
{
Console.WriteLine("Empty all the way down");
}
Code language: C# (cs)
This correctly finds the four empty subdirectories and outputs the following:
Finding all empty directories in C:\temp\EmptyTest\
Empty: C:\temp\EmptyTest\B-NotEmpty\BB-Empty
Empty: C:\temp\EmptyTest\C-Empty
Empty: C:\temp\EmptyTest\D-Empty\DD-Empty
Empty: C:\temp\EmptyTest\D-Empty
Code language: plaintext (plaintext)
The root directory is not empty. It contains at least one subdirectory that has files, so by definition, it’s not empty.